Using PowerShell to Export a Hyper-V Configuration
I'm assuming that if you are running Hyper-V in a production environment, then you probably have invested in a backup solution. What I want to demonstrate in this article isn't intended to replace those products, but rather supplement them. If you run a smaller shop, a lab environment, or client Hyper-V on a Windows 8 or later desktop, then this article may be especially handy.
The problem is that when you use the Export-VM cmdlet, you get everything and given the size of the virtual machine hard drives and snapshots, this process may take some time to complete. But perhaps you only want to export the configuration itself? I was working on this problem when I came across someone with this exact issue. He wanted to export the virtual machine configuration so that he could import it later.
The configuration that you see when you run Get-VM or look at a virtual machine settings in Hyper-V Manager are stored in an XML file. The file location is included in the virtual machine object.

The name of the file is the same as the virtual machine's id.

It is pretty easy to get that file with PowerShell.

The file should exist in a sub-folder called Virtual Machines, but sometimes it is not. There is also a possibility there might be multiple XML files, so I do this to get the full file name.

Next, I need to create the destination folder and copy the xml file to it.

In terms of a quick and easy export or backup that's all there is to it. But I'm always thinking about what other requirement someone might have. Assuming you might import the configuration file, it might be helpful to provide a new name for the virtual machine. Or remove hard drive references so that if you import on a different Hyper-V host you don't get ugly errors. Or remove snapshot references. Plus, you most likely want to do this from the comfort of your desktop. So I created a PowerShell function called Export-VMConfiguration.
Microsoft’s View on Hyper-Convergence
The traditional deployment of a vSphere or Hyper-V farm has several tiers connected by fabrics. The below diagram shows a more traditional deployment using a storage area network (SAN). In this architecture you have:
Storage trays
Switches (iSCSI or fiber channel)
Storage controllers
Virtualization hosts (application servers)
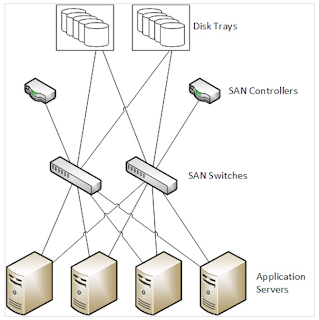
In the Hyper-V world, we are able to do a software-defined alternative to a SAN called a Scale-Out File Server (SOFS), where:
RAID is replaced by Storage Spaces
Storage controllers are replaced by a Windows Server transparent failover cluster
iSCSI and fiber channel are replaced by SMB 3.0
But for the most part, the high-level architecture doesn't really change:
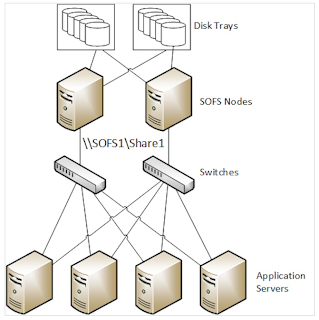
In the world of hyper-convergence, we simplify the entire architecture to a single tier of servers/appliances that run:
Virtualization
Storage/cluster network
Storage
I say "simplify" but under the covers, each server will be running like a hamster on a wheel … on an illicit hyper-stimulant.
What Microsoft Says About Hyper-Convergence
Hyper-convergence is a topic that has only been getting headlines for the last year or so. This is mainly thanks to one vendor that is marketing like crazy, and VMware promoting their vSAN solution. I had not heard Microsoft share a public opinion about hyper-convergence until TechEd Europe 2014 in October.
The message was clear: Microsoft does not think hyper-convergence is good. There are a few reasons:
The method of growing a hyper-converged infrastructure is to deploy another appliance, adding storage, memory, processor, and license requirements. This is perfect if your storage and compute needs grow at the same scale, but this is about as rare as gold laying geese. Storage requirements normally grow much faster than the need for compute. We are generating more information every day and keeping it longer. Adding the costs of compute and associated licensing when we could be instead growing an affordable storage tier makes no sense.
Performance: Hyper-converged storage is software defined. Software-defined storage, when operated at scale, requires compute power to manage the storage and perform maintenance (such as a restore after a disk failure). Which do you prioritize in a converged infrastructure: virtual machine computational needs, or the functions of storage management, which in turn affect the performance of those same virtual machines?
This is why Microsoft continues to push solutions based on a storage tier and a compute tier, which are connected by converged networks, preferably leveraging hardware offloads (such as RDMA) and SMB 3.0. The recently launched Dell/Microsoft CPS is such a solution.
6 Hyper-V Predictions for 2015
1. Docker Hype
We're going to be hearing a lot about a technology called Docker. This snowball started to roll in recent months when Microsoft announced Azure support for Docker via a partnership with the company. Docker can work in Azure right now through a command-line interface (CLI) that was launched for Windows clients, and we know that Docker is coming to Windows Server in the future.
Docker is more so an addition to Azure or Hyper-V, rather than being viewed as a distinct competitor. In machine virtualization we have isolation between machines, with each machine having its own operating system. This security boundary contains an OS, libraries, and typically one application. Containerization is done on a per application deployment basis. A Docker engine runs on a single operating system. Containers for different applications are deployed on shared libraries that are hosted by this shared operating system. The Docker engine could be installed on a physical server, or it could be run in the guest OS of a virtual machine (Azure or Hyper-V).
The benefit of Docker is that you can deploy applications nearly instantly from a library of containerized apps. This is a great benefit in a private deployment where change is frequent, such as in a DevOps environment. For example, a farm of a few virtual machines could run lots of application instances.
I think we are going to hear a lot about Docker in 2015, with the trickle becoming a torrent. But I don't think many IT pros will use Docker in 2015, as it will be early days, and I think Docker's market is geared toward larger organizations that demand a pace of change that even a flexible private/public cloud can offer.
2. Windows Server Licensing Will Switch to Per-Core
At the moment, we purchase Windows Server (and therefore Hyper-V virtual machines running Windows Server) on a per-physical processor basis. Each Windows Server license covers two physical processors, no matter how many cores are in each processor. If you license a physical host with two Intel E5-2699 v3 processors (18 cores each = 32 total cores or 64 logical processors), then you can license that host for an unlimited number of virtual machines with just one copy of Windows Server Datacenter Edition, which costs $6,155 with Open NL licensing. Remember that this license can be installed on the host and you can enable Hyper-V for free.
Here's the issue: Just a year ago, you most likely required four processors and two datacenter licenses to get a host with that sort of core density. Microsoft would have made $12,310 on Open NL license fees. Intel continues to increase the core density of hosts, and this is reducing Microsoft's earning power in larger host deployments.
I believe that Microsoft will switch from a per-pair of processors licensing model to a per-core pricing system with the release of Windows Server vNext. I can understand why because newer processors will reduce their earnings. Hopefully Microsoft will calculate the per-core pricing based on the typical processor (6 -8 cores) that most of us still use. This would mean that we wouldn't necessarily see a price rise
3. Windows Server vNext Release Details
I could be lazy and tell you that Windows Server vNext will arrive in the second half of 2015, but everyone in the business knows that. Instead, I will take some chances. I am guess-timating that general availability (not RTM) of Windows Server will be in September.
Microsoft typically uses the same naming system as games by EA Sports. The reasoning for this is simple — Microsoft's financial year starts in July, so anything released after July uses the following year. The next version of Windows Server will be released in Microsoft's financial year of 2016 and will be called Windows Server 2016.
4. Microsoft Azure Momentum
If you attend any Microsoft events or you have any dealings with a Microsoft employee, then expect to hear a lot about Azure in the first six months of 2015. Microsoft is going to be doing a lot get you hooked on their public cloud. Microsoft wants customers to try out their public and hybrid offerings. If you've got an on-premises environment, then they'll be making big pushes with online backup and disaster recovery in the cloud. Services such as site-to-site VPN and ExpressRoute have had recent upgrades, and the number of WAN partners will continue to grow. Microsoft Ignite (the super conference in Chicago in May 2015) will seem like a great big cloud conference!
No matter what you might think of the public cloud (and I was a skeptic of Microsoft's offerings!) you cannot get over:
The pricing
The incredible rate of improvement of their infrastructure-as-a-service (IaaS) offerings
The hybrid offerings that supplement (not replace) on-premises hardware.
A lot more IT pros will be managing some services in Azure by the end of 2015 than at the end of 2014, but the focus will remain on hybrid cloud.
5. You Still Won't Be Using PowerShell
This is a depressing prediction by me. Most of you reading this article will not be using PowerShell to simplify management, deployment, and operations and enable automation. I am not a programmer, but I've taken to PowerShell, where I used it to drive the demos from my presentation at TechEd Europe 2014. I use PowerShell to rebuild my demo lab at work. I use PowerShell scripts to provision large numbers of virtual machines in Hyper-V or Azure. And I use PowerShell to get predictable and fast results. There was a certain time investment to get all that stuff right, but as all scripters know, we start off by blatantly copying and pasting from other people's work from the many available online resources and tweak from there. The investment, even in small deployments, is recouped later on when that script, a function, or a cmdlet is reused over and again.
But no matter what I say, no matter what Jeffrey Snover says, and no matter what anyone in Microsoft says, most of you will ignore our advice on using PowerShell and will continue to use a subset of the product that they have purchased by not adventuring beyond the GUI.
6. Hyper-Convergence Was the Rubik's Cube of 2014
A number of hyper-convergence specialist companies have been shouting very loudly and very expensively about the benefits of their kind of compute and storage deployment. I get the feeling (my opinion and not the official view of Petri.com) that these companies are more successful at getting investment and the attention of the media than at selling their products.
I think hyper-convergence will go the way of tab, parachute pants, leg warmers, Furby, the Rubik's Cube, and music on MTV, and we'll get back to operating compute on one tier and storage on another, connected by a high-speed fabric.
How Do I Manage Hyper-V?
Just like with other virtualization and cloud platforms, there are many ways to manage Hyper-V. The choices depend on how big your installation is, what licensing you have purchased, how much automation you want to use, and if you have leveraged self-service. I will explain different administration options that are available from Microsoft in this article. Please note that other companies such as 5nine and Veeam also offer administration tools for Hyper-V.
Hyper-V Management Basics for Small to Medium Deployment
Hyper-V Manager is the basic administration tool that is included in Windows Server and Windows 8/8.1 Pro/Enterprise. We normally use Hyper-V Manager for the following scenarios:
- Managing a small number of hosts
- Configuring host settings
- Creating and managing virtual machines on non-clustered hosts
- Troubleshooting a host
It's typically bad practice to regularly log into hosts to manage them. You should enable Hyper-V Manager on your PC to manage your hosts. Not only is this a better practice, but it also makes administration quicker and easier. If you're working in a business that's using old technologies, such as Windows 7, then you can deploy a Windows Server 2012 RDS server, install Hyper-V Manager on it, and publish the application to Hyper-V administrators. This makes deploying Windows 8.1 for IT seem much easier and more economical.
Using Hyper-V Manager to control host settings.
Note: You must use a version of Hyper-V Manager that is compatible with the hypervisor version. For example, you cannot use Hyper-V Manager for Windows Server 2008 R2 to manage Windows Server 2012, as there is a mismatch of WMI versions. However, you can use the Hyper-V Manager in Windows 8.1 to manage Windows Server 2012 Hyper-V.
Highly Available Virtual Machines
If you deploy clustered hosts, then you will actually need to use two tools. If you want a virtual machine to be highly available (HA), then you will deploy and configure that virtual machine using Failover Cluster Manager. Similar to Hyper-V Manager, you should not use Failover Cluster Manager on the hosts. Instead, you should install it on your PC.
Unlike Hyper-V Manager, you will have to download the Remote Server Administration Toolkit for your version of the Windows client OS. Note that you must run Windows 8.1 on your PC to remotely manage Windows Server 2012 R2, Windows 8.1 or 8 to manage Windows Server 2012, and Windows 7 to manage Windows Server 2008 R2.
You will continue to use Hyper-V Manager to configure host settings, with one exception; Live Migration settings are configured in Failover Cluster Manager. Non-clustered hosts require a bit more security work to enable Live Migration, but a cluster has its own security boundary that makes Live Migration almost a click-and-go experience.
Using Failover Cluster Manager to manage highly available virtual machines.
Creating virtual machines in Hyper-V Manager on a Hyper-V cluster is a mistake that is commonly made by people that are new to Hyper-V. The resulting virtual machine is not HA, but you can fix this with the following steps:
- Using Live Storage Migration to move the virtual machines files to the cluster's storage if required.
- Using Configure Role to add the existing virtual machine to the management scope of the cluster. No restarts are required of the virtual machine.
Prior to Windows Server 2012 Hyper-V, a common mistake was to configure virtual machine settings in Hyper-V Manager. Those settings would be lost if the virtual machine moved nodes because the settings were not saved in the cluster's database. If you forget to use Failover Cluster Manager you can update the settings of the virtual machine in the cluster. Note that Windows Server 2012 prevents you from editing the settings of HA virtual machines in Hyper-V Manager.
Managing Hyper-V in Medium to Large Deployments
There are several different scenarios where you will purchase System Center with per-host licensing and use System Center Virtual Machine Manager (SCVMM) to manage Hyper-V, including:
- Scale: You need a solution to centrally manage lots of hosts/clusters.
- Cloud: You will be deploying public or private clouds.
- Deployment: You need faster host, storage, virtual machine, or service deployment.
- Delegation: You want to enable delegation of administration, enabling some administrators to manage some hosts.
Managing a cloud fabric using System Center Virtual Machine Manager (SCVMM)
Although SCVMM can be used to deploy Hyper-V clusters in theory, I still prefer to configure and manage clusters using Failover Cluster Manager, where I use SCVMM's bare metal deployment to create the hosts. I have found that SCVMM is not so hot in this department.
Note that SCVMM is just one of eight products in the System Center license suite. You will choose from the System Center menu to enable other elements of management, protection, and automation.
Hyper-V Automation
PowerShell… yes, I said it. If you want to do things at scale, get repeatable and predictable results, and do it quickly, then invest some time in Windows PowerShell to get the job done. And you'll also get access to deeper features that are not otherwise revealed in a GUI. You can use cmdlets from Hyper-V, Failover Cluster Manager, and SCVMM to write your scripts in the easy to use Integrated Scripting Editor (ISE).
Configuring Hyper-V host networking using a PowerShell script.
Using Hyper-V, System Center, and Windows Azure Pack
You will deploy a cloud whenever you want to enable self-service deployment of virtual machines and services. The front-end of a Microsoft cloud based on Hyper-V and System Center is the Windows Azure Pack (WAP). There are two portals:
- The administrative portal: Used to manage the cloud
- The tenant portal: Used by users of the cloud to deploy virtual machines and services
Note that you will continue to use System Center to manage and monitor the fabric of the cloud.
5 Hyper-V Skills
I do not argue that Hyper-V still has a smaller total market share than vSphere. But we have evidence from IDC that Hyper-V continues to grow while vSphere is shrinking. And outside of the USA, at least here in Europe, Hyper-V has a much larger presence than in the USA, possibly because we Europeans were slower to adopt virtualization. It's for this reason that IT pros should start to learn how to deploy Hyper-V… correctly.
I work for a distributor, so I am normally one-step removed from working on customer sites; I sell to the resellers, I teach the resellers, and from time-to-time, I help the resellers or my local Microsoft office out when there is an issue with a project. When I am called into a Hyper-V project it is more often than not because a VMware consulting company has been trying to deploy Hyper-V with a design that they would normally use for vSphere. My Hyper-V MVP colleagues from around Europe have a plethora of stories of when they've been called to undo the damage done in Hyper-V deployments by vSphere experts.
Let me ask you a simple question: would you use the same techniques for a gasoline engine as you would for a diesel engine? Of course not, so why would anyone assume that the way to design vSphere is universally correct? I would not assume that how I would deploy Hyper-V is universally correct. If you are going to be working with Hyper-V then you need to learn how to deploy Microsoft hypervisor correctly, including Failover Clustering, Windows Server networking, Windows Server storage, and more.
2. Learn System Center Virtual Machine Manager (SCVMM)
If you are going to deploy a larger Hyper-V farm, then you should strongly consider deploying System Center Virtual Machine Manager (SCVMM). For you vSphere folks, SCVMM is not Microsoft's answer to vCenter; it is much more than a central console for hosts and virtual machines. I believe SCVMM should be renamed to System Center Fabric Manager because it is the tool that Microsoft envisions owners of larger systems will use to manage storage and networking, deploy bare-metal hosts and Scale-Out File Servers, and provision highly-available services with deep integration into any available load balancers.
In my experience, SCVMM is underused by those customers who do buy System Center licensing. Yes, Hyper-V Manager, Failover Cluster Manager, and PowerShell can do a lot, but SCVMM brings more to the table, especially in environments where change is frequent. There are three kinds of Hyper-V customers who buy System Center:
Sites that deploy other parts of System Center, typically SCOM and SCCM, but not SCVMM and miss out on lots of functionality.
Customers that do have SCVMM installed, but use it as nothing more than a centralized Hyper-V Manager.
The minority of sites that do make great use of SCVMM.
Not everyone can afford System Center, and there are those that have concerns with the complexity of SCVMM. But for those of you that do have that licensing, my advice is that you learn the tool and make better use of it. If you are in the role of service delivery (internal or external customers), then SCVMM is the starting point for build a Windows Azure Pack (WAP) powered private or public cloud.
3. Understand SMB 3.0
If you read my posts then it should be no surprise that I am advocate of using Scale-Out File Server (SOFS) storage for Hyper-V virtual machines. SOFS was the first technology to make real use of Microsoft strategic data transmission protocol, SMB 3.0. In the role of storage connectivity between hosts and a file share, SMB 3.0 competes against the likes of iSCSI, fiber channel and fiber channel over Ethernet (FCoE). However, Microsoft had bigger plans for SMB 3.0. In Windows Server 2012 R2, SMB 3.0 can be used for Live Migrations running at speeds of 10 Gbps or faster. In Windows Server vNext, SMB 3.0 expands again for synchronous and asynchronous replication between Windows servers.
SMB 3.0 is here to stay. There are three components or concepts you need to learn:
- SMB Multichannel: What NICs are selected and how to control this
- SMB convergence: How to design converged networks with bandwidth controls
- SMB Direct: You are going to be pushing huge amounts of data, so you need to learn about hardware offloading via Remote Direct Memory Access (RDMA) via iWarp, RoCE, or Infiniband
For those of you running more than a few hosts, you need to release that death grip on 1 GbE networking. 10 GbE is here, and for some of you, you need to be looking at 40 Gbps, 56 Gbps, or even faster! This isn't because of Hyper-V; this is because you are creating larger virtual machines, generating more data, and retaining data for longer than ever before. Stuff needs to move across networks, and it needs to happen quickly.
This typically requires investing in expensive switches, where the NICs don't actually have to be that expensive if you shop wisely, so you need to make the very most of every switch port. This is why we deploy converged networking, once the reserve of hardware solutions, such as those found in blade server enclosures.
Right now, many people are deploying Hyper-V hosts with lots of 1 GbE NICs, or they're using very expensive converged-networking hardware. Those who use 1 GbE networking have bandwidth limitations for individual services or virtual machines. And most of those who are using Windows Server 2012 R2 on blade servers have grown frustrated with Emulex, who have near-monopoly status in this market, taking a year to resolve outage issues with the firmware and drivers of their converged networking NICs.
I have been an advocate of using the features of converged networking that are free in Windows Server. Place a pair of 10 GbE or faster NICs into your host, team them, create a Hyper-V virtual switch that connects to that team, and connect the host and virtual machines to ports on the virtual switch. Now the host and virtual machines have access to 10 Gbps networking while consuming just two top-of-rack (TOR) switch ports. Don't get caught up in CAPEX costs. Remember that the cost of powering six to 10 x 1 GbE posts per host is probably much more than powering two x 10 GbE switch ports per host. OPEX cost savings over three years must be accounted for, too!
4. Embrace Microsoft Azure
2015 will be the year that you finally have to admit that you're going to do some stuff in the cloud. I came to that realization in early 2014 after very successfully ignoring Office 365 and Microsoft Azure for a long time. Your bosses are going to be hearing a lot in the media and directly from Microsoft about the cloud. Pressure will build, and if you won't do it, they'll find someone who will.
There are easy ways to embrace the cloud to supplement your on-premises environment. Everyone struggles with the costs of disaster recovery. Maybe Azure Site Recovery will give you an reasonably-priced solution for disaster recovery in the cloud? I hated working with tapes and tape drives, so maybe backup to Azure will service your needs for off-site backup and archiving? Do you need a hybrid web presence? If so, check out Azure website hosting plans, starting with free offerings. Or do you need a test/dev lab for IT or for the developers and testers? If so, why spends money on hardware when you can pay for what you use in Azure with supervision and enforcement by IT?
5. Learn PowerShell
You're using just 40% of the product you've invested in if you refuse to consider using PowerShell. Most of the magic that I show and talk about is driven by PowerShell and does not have a UI. I guarantee you that this percentage will decrease in the next version of Hyper-V. If you worked for me, I'd fire you. Harsh words, but sometimes a kick in the rear is required.
2015 will be a year of disruption in the world of Hyper-V. There will be the release of Windows Server vNext in the second half of the year. Azure will be purchased by more and more CIOs, with or without the cooperation of their IT departments, but there'll be no choice about deploying services in the public cloud. And storage and networking will continue to evolve. You can prepare yourself for a very interesting year by spending some time learning the above technologies.